REST request
You can add an HTTP REST request to your service in the Info View. When the listener chooses the HTTP request action, Sonos sends an HTTP request using your given URL and HTTP method. Your service's response back to Sonos can be a simple success response, an error response, or a more complex response that Sonos uses to modify the custom actions displayed in the Info View.
Supported scheme
The only supported scheme to use for the URL is secure HTTP,
https://
.
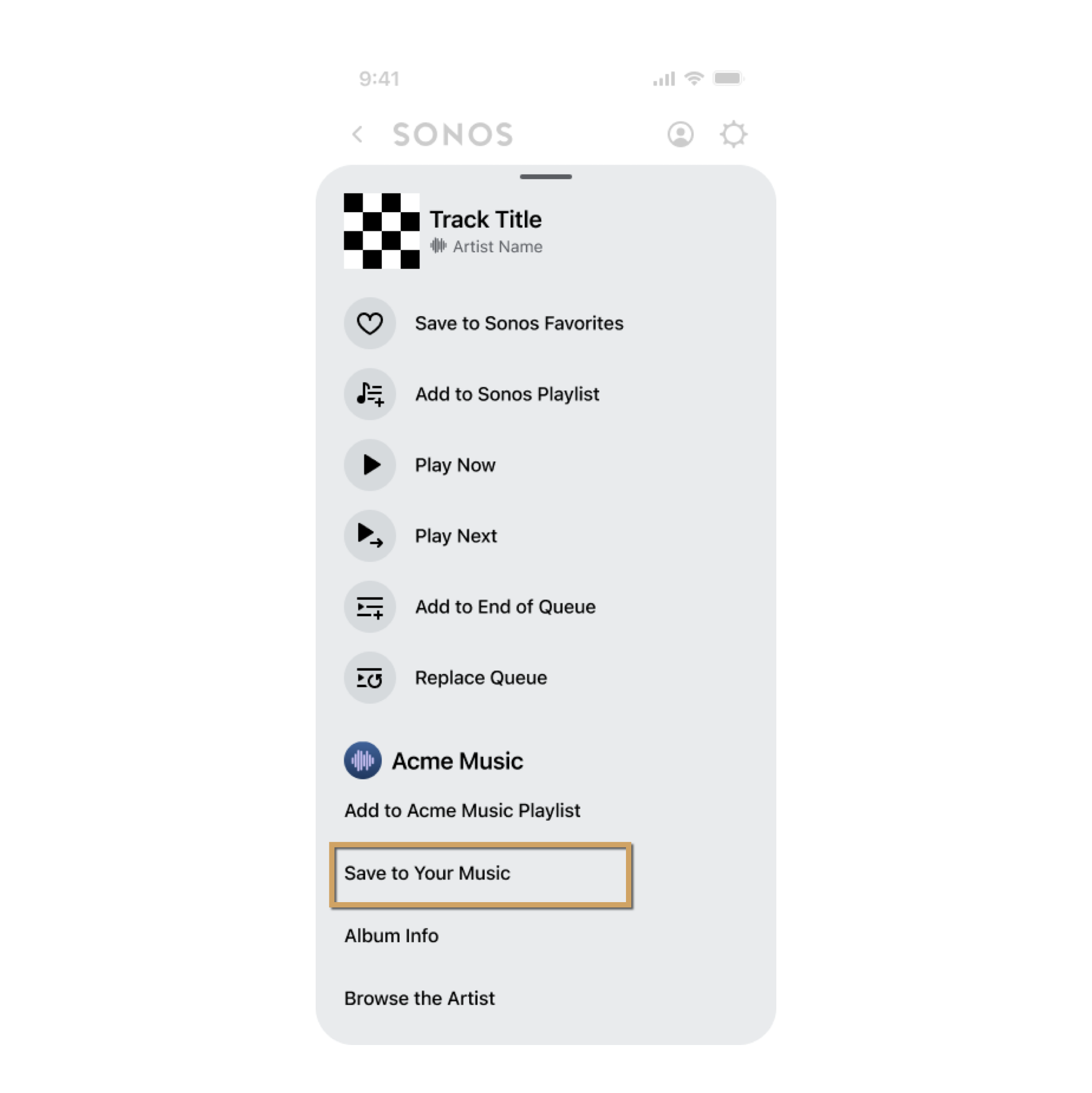
The example in this section shows how to save an item to your service's favorites. For a complete favorites implementation, see Add favorites.
The following steps describe how to implement an HTTP request action.
- Define text strings for the HTTP request action.
- Respond to getExtendedMetadata requests from Sonos.
- Implement the REST method on your service.
- Respond back to Sonos for the HTTP request.
Define HTTP request action text strings
Define the string in your i18next file that displays for the HTTP request action in the Info View of the Sonos app. For this example we use, "Save to My Acme Music". Also define any error strings that might be needed.
{
"en-US":{
"common":{
...
},
"integration":{
"SAVE_MUSIC": "Save to My Acme Music",
"REMOVE_MUSIC": "Remove from My Acme Music",
"SAVE_SUCCESS": "Saved to your Acme Music favorites",
"SAVE_FAILED": "Failed to save to your Acme Music favorites"
...
}
},
}
Respond to getExtendedMetadata requests
When the listener selects the ellipsis (...) to see the Info View, Sonos sends your service a getExtendedMetadata request. This example is for a track item.
<s:Envelope
xmlns:s="<http://schemas.xmlsoap.org/soap/envelope/>">
<s:Header>
...
</s:Header>
<s:Body>
<getExtendedMetadata xmlns="http://www.sonos.com/Services/1.1">
<id>tr:15</id>
</getExtendedMetadata>
</s:Body>
</s:Envelope>
When your service responses to getExtendedMetadata, include all the custom action items for the Info View. To simplify this example we'll show only one HTTP request.
<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Body>
<getExtendedMetadataResponse xmlns="http://www.sonos.com/Services/1.1">
<getExtendedMetadataResult>
<mediaMetadata>
<id>tr:15</id>
<itemType>track</itemType>
<title>Man in the Mirror (2003 Edit)</title>
<mimeType>audio/mp3</mimeType>
<trackMetadata>
<artist>Michael Jackson</artist>
<composer/>
<album>Number Ones</album>
<genre>80s Pop</genre>
<duration>303</duration>
<albumArtURI>http://cdn.acme.com/fYpMChhFbjwT0yA</albumArtURI>
<canPlay>true</canPlay>
</trackMetadata>
</mediaMetadata>
<relatedActions>
<action>
<id>add_track_to_favorites_id</id>
<title>SAVE_MUSIC</title>
<actionType>simpleHttpRequest</actionType>
<simpleHttpRequestAction>
<url>https://192.168.0.10:8223/v2/favorites?trackId=tr:15</url>
<method>PUT</method>
<httpHeaders>
<httpHeader>
<header>clientid</header>
<value>sonos-to-acme-client</value>
</httpHeader>
</httpHeaders>
<refreshOnSuccess>true</refreshOnSuccess>
</simpleHttpRequestAction>
<showInBrowse>true</showInBrowse>
<failureMessageStringId>SAVE_FAILED</failureMessageStringId>
</action>
<action>
. . . Details of other actions in the Info View are not shown . . .
</action>
</relatedActions>
. . . Details for other custom actions in the Info View are not shown . . .
</getExtendedMetadataResult>
</getExtendedMetadataResponse>
</soap:Body>
</soap:Envelope>
The action
sub-elements for an HTTP request action include the following. See SMAPI object types for details.
Element | Description |
---|---|
actionType | This value for an HTTP request action must be simpleHttpRequest . |
id | A unique ID for this action. |
title | The SAVE_MUSIC value corresponds to the stringId value in the i18next file above. |
simpleHttpRequestAction | This contains the following sub-elements:url – the URL of the HTTP request Sonos will send if the listener selects this HTTP request action from the Info View.method – The HTTP method to execute. Valid values are the standard methods POST , GET , PUT , or DELETE .httpHeaders (Optional) – Custom HTTP headers can be added to the HTTP request by including name/value pairs in httpHeader sub-elements.refreshOnSuccess (Optional) – Include a value of true to cause Sonos to update the container the listener is browsing after the HTML request successfully executes. |
failureMessageStringId successMessageStringId | Optional. You can supply stringIdvalues for failureMessageStringId and successMessageStringId that Sonos displays to the listener depending on the execution results of the HTTP request.Note that values for these can be overwritten by returning failureMessageStringId and successMessageStringId JSON values in your response back to Sonos for the HTTP REST request. |
Implement the REST method on your service
If the listener chooses the HTTP request action from the Info View, Sonos sends the HTTP request using the given method and URL. Sonos includes the current SMAPI authentication token in an Authorization
header as an OAuth Bearer token. Note, you cannot use custom httpHeaders
to attempt to overwrite the Authorization
header. For more about authentication, authorization, and tokens, see Use authentication tokens.
The following is an example HTTP REST request from Sonos:
PUT <https://192.168.0.10:8223/v2/favorites?trackId=tr:15>
Host: music.acme.com
clientid: sonos-to-acme-client
Authorization: Bearer 614e6c9ce07be03ece09a8639ea40dcbd
Your service provides the implementation. For this example you would add the track to this listener's favorites list on your service.
Respond back to Sonos for the HTTP request
In your response to the HTTP request back to Sonos, you can supply optional string IDs in JSON format that identify messages Sonos displays to the listener depending on the success or failure execution of the HTTP request. You can also supply more complex JSON objects that Sonos uses to modify the Info View the listener sees.
Success responses
For a basic success response, you can supply an optional stringId
value in a successMessageStringId
JSON object that identifies a string in your i18next file.
If you return
successMessageStringId
here, it overrides the value if previously supplied in thesuccessMessageStringId
XML element in your original getExtendedMetadata response that set up the HTTP request.
A simple JSON success response back to the Sonos app might look like this:
{
"status": 200,
"successMessageStringId": "SAVE_SUCCESS"
}
Failure responses
You can supply an optional stringId
value in a failureMessageStringId
JSON object that identifies a string in your i18next file.
If you return
failureMessageStringId
here, it overrides the value if previously supplied in thefailureMessageStringId
XML element in your originalgetExtendedMetadata
response that set up the HTTP request.
A JSON failure example response back to the Sonos app might look like the following:
{
"status": 500,
"failureMessageStringId": "SAVE_FAILED"
}
If the HTTP request results in a 401 error to Sonos, this may indicate that the authentication token has expired. Sonos will attempt to refresh the token by making a refreshAuthToken request to your service. If the token refresh succeeds, then Sonos will retry the original HTTP request with the new token in the Authorization
header. Sonos will only retry the HTTP request once. If it fails again, Sonos will display the failure message. See Use authentication tokens for details.
Responses to change the Info View
To modify the listener's Info View after the HTTP request, send a more complex JSON response to Sonos. For example, after adding a track to the listener's favorites, you might want to change the "Save to My Acme Music" action to be the action "Remove from My Acme Music", as shown below.
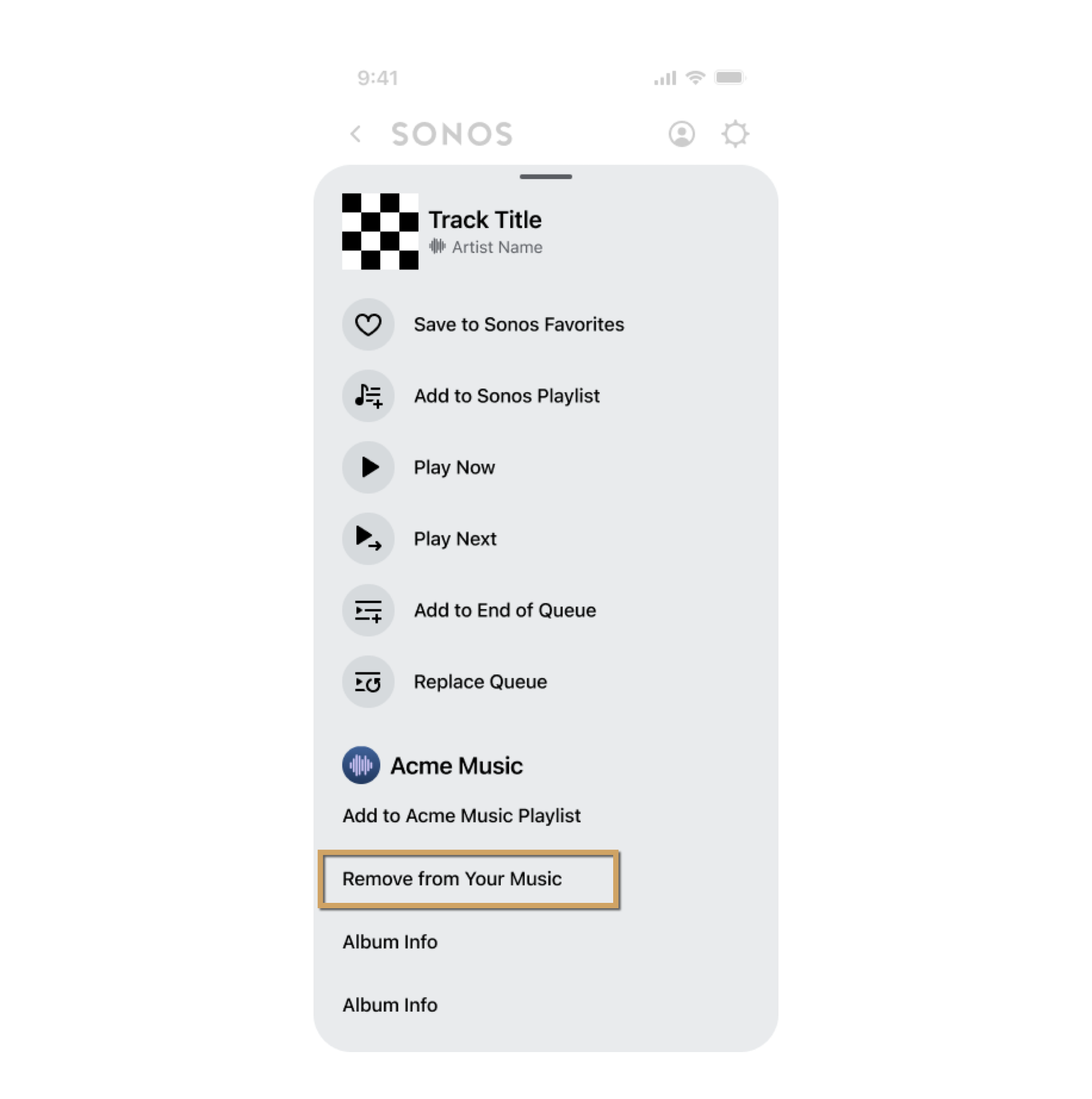
In the JSON response, return all of the actions that you want in the relatedActions
object. The screen above shows two related actions:
- An HTTP request action, "Remove from My Acme Music", implemented with a
simpleHttpRequestAction
object in the JSON response. - An open URL action, "Concerts", implemented with an
openUrlAction
object in the JSON response. See Webpage for details.
All other custom actions in the Info View remain unchanged. Following is the associated JSON response.
HTTP/1.1 201
Content-Type: application/json;charset=UTF-8
Transfer-Encoding: chunked
Connection: close
{
"relatedActions": [
{
"actionType": "simpleHttpRequest",
"id": "remove_track_from_favorites",
"title": "REMOVE_MUSIC",
"simpleHttpRequestAction": {
"httpHeaders": [
{
"name": "clientid",
"value": "sonos-to-acme-client"
}
],
"method": "DELETE",
"refreshOnSuccess": true,
"url": "<https://192.168.0.10:8223/v2/favorites?trackId=tr:15>"
},
"showInBrowse": true,
"successMessageStringId": "HTTP_REQUEST_DELETE_SUCCESS",
"failureMessageStringId": "REMOVE_FAILED"
},
{
"actionType": "openUrl",
"id": "concerts_action_id",
"title": "CONCERTS_STRING",
"openUrlAction": {
"url": "<https://www.acme.com/concerts/?artistId=a:12345678&tracker=foo>"
},
"showInBrowse": true
}
],
"successMessageStringId": "DELETE_SUCCESS"
}
For details of the relatedActions
JSON array, see SMAPI object types.
Updated about 1 year ago